How to Sort an Array or List of Numbers in Java
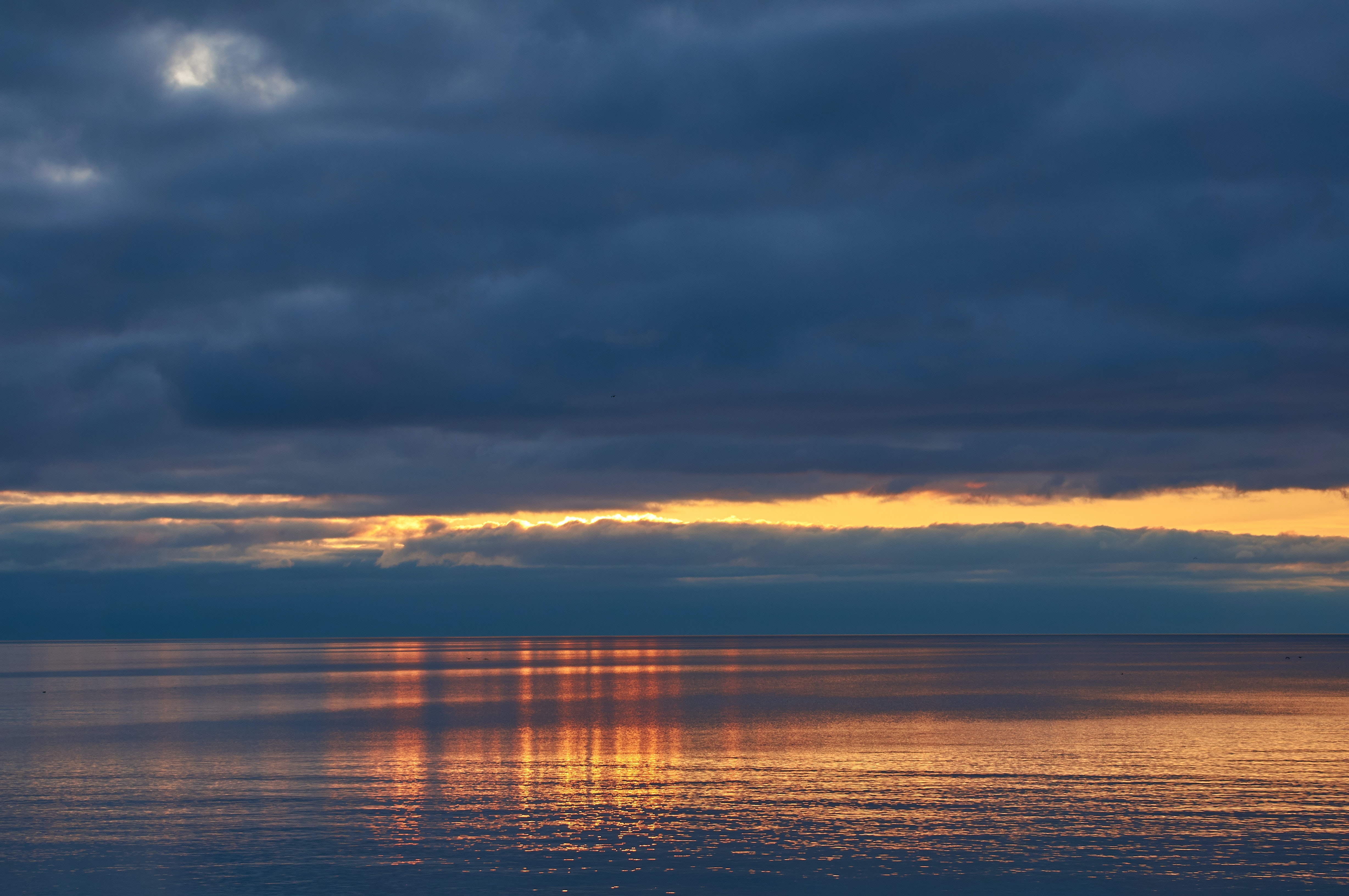
Overview
1. Introduction
In this post, you'll learn how to sort in-place lists and arrays of numbers. Sorting in place means we don't need to create another list or array. We sort the input in the same variable.
2. Sort a List of Number Wrapper Classes
We can use sort from the List interface and some methods from the Comparator interface to list arrays or lists in place. The sort method takes a Comparator interface as an argument. That comparator will tell the sort method which order to use.
With the methods described, you can sort lists of any numeric wrapper class, for example, Integer, Double, and Float. Since sort is an instance method of List, that will not work with collections of primitive types.
2.1. Sort a List of Integer in Ascending Order
To sort in ascending order, let's create a Comparator using the static method naturalOrder and pass it to the sort method:
1 public static void main(String[] args) {
2 List<Integer> collection1 = Arrays.asList(-1, 2, 0, -10, 4, 5);
3 collection1.sort(Comparator.naturalOrder());
4 System.out.println(collection1); // -> [-10, -1, 0, 2, 4, 5]
5 }
Note: The Comparator.naturalOrder is the default value of the sort. The method sorts in ascending order when you don't pass any argument to it.
2.2. Sort a List of Integer in Descending Order
To sort in descending order, let's use the reverseOrder instead of naturalOrder.
1 public static void main(String[] args) {
2 List<Integer> collection2 = Arrays.asList(-1, 2, 0, -10, 4, 5);
3 collection2.sort(Comparator.reverseOrder());
4 System.out.println(collection2); // -> [5, 4, 2, 0, -1, -10]
5 }
2.3. Sort a List of Integer using Nulls First or Last Strategy
Sorting by null is sometimes required when null means something in your application, although there are better practices than this.
The Integer class accepts null as a valid value. It's possible to compare null and numbers using the nullsFirst() or nullsLast() methods. Those two methods return another Comparator, so we can keep concatenating new static methods to create a more specific comparator.
Let's see one example of sort placing nulls first and then using ascending order:
1 public static void main(String[] args) {
2 List<Integer> collection3 = Arrays.asList(-1, 2, 0, null, -10, 4, 5, null);
3 collection3.sort(Comparator.nullsFirst(Comparator.naturalOrder()));
4 System.out.println(collection3); // -> [null, null, -10, -1, 0, 2, 4, 5]
5 }
To place nulls last, we can use the nullsLast method:
1 public static void main(String[] args) {
2 List<Integer> collection3 = Arrays.asList(-1, 2, 0, null, -10, 4, 5, null);
3 collection3.sort(Comparator.nullsLast(Comparator.naturalOrder()));
4 System.out.println(collection3); // -> [-10, -1, 0, 2, 4, 5, null, null]
5 }
3. Sort an Array of Primitive Values
Working with primitive types is slightly different compared to numeric wrapper classes. Here you can use the sort from Arrays and pass the array as an argument.
Note that nulls aren't allowed in an array of primitives
3.1. Sort an Array of int in Ascending Order
It is straightforward to sort an array of ints using sort. Let's see that in practice:
1 public static void main(String[] args) {
2 int[] collection5 = new int[]{-1, 2, 0, -10, 4, 5};
3 Arrays.sort(collection5);
4 System.out.println(Arrays.toString(collection5)); // -> [-10, -1, 0, 2, 4, 5]
5 }
In the example, we passed the array as an argument of the sort method, which is sufficient to sort the array.
3.2. Sort an Array of int in Descending Order
There's no suitable method in the JDK to sort an array of primitives in place in decreasing order. One way to do that is to box the primitive type to the Integer class, sort the array using a reverse order comparator, then map the values back to their primitive type. Let's see that in practice:
1 public static void main(String[] args) {
2 int[] collection6 = new int[]{-1, 2, 0, -10, 4, 5};
3
4 int[] sorted = IntStream.of(collection6)
5 .boxed()
6 .sorted(Collections.reverseOrder())
7 .mapToInt(Integer::intValue)
8 .toArray();
9
10 System.out.println(sorted); // -> [5, 4, 2, 0, -1, -10]
11 }
The of method creates a stream of primitives, which is then boxed to an Integer using the boxed method. Then, we sort the stream using sorted method and the reverOrder comparator. Finally, we collect the primitive values into an array using the mapToInt and the toArray methods.
4. Conclusion
In this article, we've seen ways to sort an array and list of primitive int and Integer classes.