Short Circuit Operators in Java
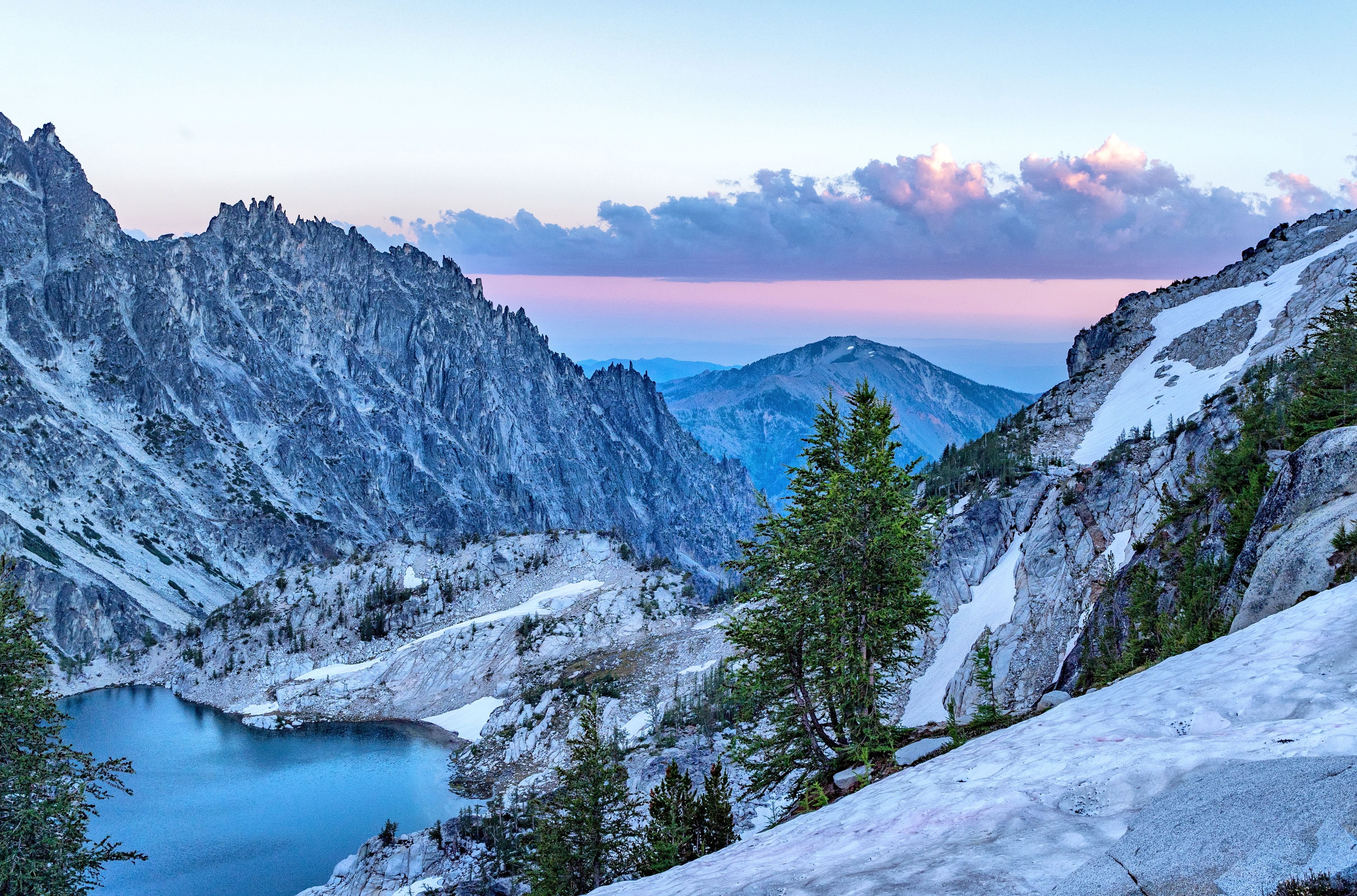
Overview
1. Introduction
In this tutorial, we'll look at the logical AND (&&) and the logical OR (||) short-circuit operators and how to use them to improve our application's performance.
2. Short-Circuit Operators
Short-circuit operators in Java are logical operators that evaluate their second operand only if the first operand does not provide a satisfactory result to resolve the overall expression.
They are typically valuable to optimize conditionals by optimizing unnecessary evaluations. Evaluating the first logical OR (||) operand to true makes it entirely true. While the logical AND (&&) makes the entire expression false if the first operand is false.
3. The AND Operator
Understanding the logical AND truth table helps to comprehend short-circuit operators. Suppose that P and Q are operands of our conditional statements. The result of P AND Q is displayed below:
P | Q | P AND Q |
---|---|---|
True | True | True |
True | False | False |
False | True | False |
False | False | False |
The AND operator evaluates to true only when both operands are true. Therefore, if the first operand is false, then the only possible outcome for the entire expression is false.
Let's have a look at how to short-circuit the AND operator:
1public class ShortCircuitAndExample {
2
3 public static void main(String[] args) {
4
5 // |-----------------------| Evaluates up to here
6 if (isEven(10) && isEven(6)) {
7 System.out.println("Evaluating both expressions, since the first is true!");
8 }
9
10 // |--------| Evaluates up to here
11 if (isEven(7) && isEven(8)) {
12 System.out.println("Evaluating just the first expression, since it is false!");
13 }
14 }
15
16 private static boolean isEven(int number) {
17 return number % 2 == 0;
18 }
19}
In the first if statement, the call to isEven(10) is true, so isEven(6) must be evaluated to obtain the final result.
In the second if statement, the call to isEven(7) is false, which evaluates the whole expression to false according to the AND truth table. Thus the JVM skips the call to isEven(8).
4. The OR Operator
To illustrate how the logical OR works, let's look at its truth table:
P | Q | P OR Q |
---|---|---|
True | True | True |
True | False | True |
False | True | True |
False | False | False |
The OR operator only evaluates to false when both expressions are false. Thus, if any operand is true, it makes the entire expression true. Therefore, we can short-circuit the logical OR if the first operand is true, skipping any other operands after that.
Let's see how that works using a Java example:
1public class ShortCircuitOrExample {
2
3 public static void main(String[] args) {
4
5 // |---------| Evaluates up to here
6 if (isEven(10) || isEven(6)) {
7 System.out.println("Evaluating just the first expression, since it is true!");
8 }
9
10 // |----------------------| Evaluates up to here
11 if (isEven(7) || isEven(8)) {
12 System.out.println("Evaluating both expressions, since the first is false!");
13 }
14 }
15
16 private static boolean isEven(int number) {
17 return number % 2 == 0;
18 }
19}
In the first if statement, the JVM evaluates only the call to isEven(10) and skips the other operands. Since isEven(10) is true, the entire expression is true, regardless of the result of the following operands.
In the second if statement, both operands isEven(7) and isEven(8) need to be evaluated because isEven(7) is false.
5. Code Optimization and null Checking
The typical applications for short-circuit operators are to optimize code and to make the code null safe.
Regarding code optimization, we should build the expression so that the operands are ordered in terms of cheapness for their computer resource usage (time, RAM, disk, etc.). For instance, suppose an if statement is composed of the three operands: a call to isEven, a list traversal, and an HTTP call. In that case, the correct order of operands should be: 1. isEven, 2. the list traversal, 3. HTTP call.
About null checking, we should always keep the null check operand at first together with a logical AND. By doing that, we guarantee that we'll never use a null reference in the following operands. For instance, suppose we have a nullable list variable named myList, and we need to use its first elements using get. The null-safe if statement is like follows:
1if (myList != null && myList.get(0) == 2) {
2 //do something
3}
6. Conclusion
In this article, we've seen the two short-circuit operators in Java (&& and ||) and how to use them to make our code safer and faster.